Spring AOP面向切面編程實現原理方法詳解
1. 什么是AOP
AOP (Aspect Oriented Programming)意為:面向切面編程,通過預編譯方式和運行期動態代理實現在不修改源代碼的情況下,給程序動態統一添加功能的一種技術,可以理解成動態代理。是Spring框架中的一個重要內容。利用 AOP 可以對業務邏輯的各個部分進行隔離,使業務邏輯各部分之間的耦合度降低,提高程序的可重用性,同時提高開發的效率
2. Spring AOP
①. AOP 在Spring中的作用
提供聲明式事務;允許用戶自定義切面
②. AOP 的基本概念
橫切關注點:跨越應用程序多個模塊的方法或功能。即與我們業務邏輯無關,但需要我們關注的部分就是橫切關注點。如日志 , 安全 , 緩存 , 事務等等 ....
Aspect(切面):橫切關注點被模塊化的特殊對象。通常是一個類,里面可以定義切入點和通知 Weaving(織入):把切面(aspect)連接到其它的應用程序類型或者對象上,并創建一個被通知(advised)的對象。 這些可以在編譯時,類加載時和運行時完成。Spring和其它純Java AOP框架一樣,在運行時完成織入 Advice(通知):AOP在特定的切入點上執行的增強處理,是切面必須要完成的工作,也是類中的一個方法 Target(目標):被通知對象 AOP(代理):AOP框架創建的對象,代理就是目標對象的加強。Spring中的 AOP 代理可以是 JDK 動態代理,也可以是 CGLIB 代理,前者基于接口,后者基于子類 JointPoint(連接點):程序執行過程中明確的點,一般是方法的調用 Pointcut(切入點):就是帶有通知的連接點,與切入點匹配的執行點③. 使用Spring實現Aop
前提
使用AOP織入,需要導入一個依賴包
<dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.9.5</version></dependency>
實現Aop的三種方式
方式一:通過 Spring API 實現【主要是springAPI接口實現】
首先編寫業務接口和實現類
public interface UserService { public void add(); public void delete(); public void update(); public void search();}
public class UserServiceImpl implements UserService{ public void add() { System.out.println('增加了一個用戶'); } public void delete() { System.out.println('刪除了一個用戶'); } public void update() { System.out.println('更新了一個用戶'); } public void select() { System.out.println('查詢了一個用戶'); }}
接著編寫增強類,這里寫兩個:前置增強Log和后置增強AfterLog
import org.springframework.aop.MethodBeforeAdvice;import java.lang.reflect.Method;public class Log implements MethodBeforeAdvice { //method: 要執行的目標對象的方法 //args: 參數 //target: 目標對象 public void before(Method method, Object[] args, Object target) throws Throwable { System.out.println(target.getClass().getName()+'的'+method.getName()+'被執行了'); }}
import org.springframework.aop.AfterReturningAdvice;import java.lang.reflect.Method;public class AfterLog implements AfterReturningAdvice { //returnValue;返回值 public void afterReturning(Object returnValue, Method method, Object[] args, Object target) throws Throwable { System.out.println('執行了'+method.getName()+'方法,返回結果為:'+returnValue); }}
最后在Spring的文件中注冊( applicationContext.xml ),并實現AOP切入,注意導入約束
<?xml version='1.0' encoding='UTF-8'?><beans xmlns='http://www.springframework.org/schema/beans' xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance' xmlns:aop='http://www.springframework.org/schema/aop' xsi:schemaLocation='http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd'> <!--注冊bean--> <bean /> <bean /> <bean /> <!--方式一:使用原生Spring API接口 --> <!--配置aop:需要導入aop的約束--> <aop:config> <!--切入點:expression:表達式,execution(要執行的位置! * * * * *) --> <aop:pointcut expression='execution(* com.lf.service.UserServiceImpl.*(..))'/> <!--執行環繞; advice-ref執行方法 . pointcut-ref切入點--> <aop:advisor advice-ref='log' pointcut-ref='pointcut'/> <aop:advisor advice-ref='afterLog' pointcut-ref='pointcut'/> </aop:config></beans>
進行測試:
import com.lf.service.UserService;import org.junit.Test;import org.springframework.context.ApplicationContext;import org.springframework.context.support.ClassPathXmlApplicationContext;public class MyTest { @Test public void test(){ ApplicationContext context = new ClassPathXmlApplicationContext('applicationContext.xml'); UserService userService1 = (UserService) context.getBean('userService'); UserService userService = (UserService) context.getBean('userService'); userService.add(); }}
運行結果:
com.lf.service.UserServiceImpl的add被執行了增加了一個用戶執行了add方法,返回結果為:null
方式二:自定義類實現AOP【主要是切面定義】
目標業務類不變,還是方式一中的UserServiceImpl
寫入一個切入類
public class DiyPointCut { public void before(){ System.out.println('========方法執行前========='); } public void after(){ System.out.println('========方法執行后========='); }}
在Spring中配置(applicationContext.xml)
<?xml version='1.0' encoding='UTF-8'?><beans xmlns='http://www.springframework.org/schema/beans' xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance' xmlns:aop='http://www.springframework.org/schema/aop' xsi:schemaLocation='http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd'> <!--注冊bean--> <bean /> <bean /> <bean /> <!--方式二:自定義類--> <bean /> <aop:config> <!--自定義切面, ref 要引用的類--> <aop:aspect ref='diy'> <!--切入點--> <aop:pointcut expression='execution(* com.lf.service.UserServiceImpl.*(..))'/> <!--通知--> <aop:before method='before' pointcut-ref='point'/> <aop:after method='after' pointcut-ref='point'/> </aop:aspect> </aop:config></beans>
在上面的 MyTest.java 中測試,得到結果:
========方法執行前=========增加了一個用戶========方法執行后=========
方式三:使用注解實現【多用】
編寫一個注解實現的增強類
package com.lf.diy;import org.aspectj.lang.ProceedingJoinPoint;import org.aspectj.lang.Signature;import org.aspectj.lang.annotation.After;import org.aspectj.lang.annotation.Around;import org.aspectj.lang.annotation.Aspect;import org.aspectj.lang.annotation.Before;@Aspect //標注這個類是一個切面public class AnnotationPointCut { @Before('execution(* com.lf.service.UserServiceImpl.*(..))') public void before(){ System.out.println('=====方法執行前======'); } @After('execution(* com.lf.service.UserServiceImpl.*(..))') public void after(){ System.out.println('=====方法執行后======'); } //在環繞增強中,我們可以給定一個參數,代表我們要獲取處理切入的點; @Around('execution(* com.lf.service.UserServiceImpl.*(..))') public void around(ProceedingJoinPoint jp) throws Throwable { System.out.println('環繞前'); Signature signature = jp.getSignature();//獲得簽名 System.out.println('signature:'+signature); Object proceed = jp.proceed(); //執行方法 System.out.println('環繞后'); System.out.println(proceed); }}
在Spring配置文件中,注冊bean,并增加支持注解的配置
<?xml version='1.0' encoding='UTF-8'?><beans xmlns='http://www.springframework.org/schema/beans' xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance' xmlns:aop='http://www.springframework.org/schema/aop' xsi:schemaLocation='http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd'> <!--注冊bean--> <bean /> <bean /> <bean /> <!--方式三--> <bean /> <!--開啟注解支持! JDK(默認 proxy-target-class='false') cglib(proxy-target-class='true')--> <aop:aspectj-autoproxy/></beans>
在 MyTest.java 中測試
import com.lf.service.UserService;import org.junit.Test;import org.springframework.context.ApplicationContext;import org.springframework.context.support.ClassPathXmlApplicationContext;public class MyTest { @Test public void test(){ ApplicationContext context = new ClassPathXmlApplicationContext('applicationContext.xml'); UserService userService = (UserService) context.getBean('userService'); userService.add(); }}
得到結果:
環繞前signature:void com.lf.service.UserService.add()=====方法執行前======增加了一個用戶=====方法執行后======環繞后null
以上就是本文的全部內容,希望對大家的學習有所幫助,也希望大家多多支持好吧啦網。
相關文章:
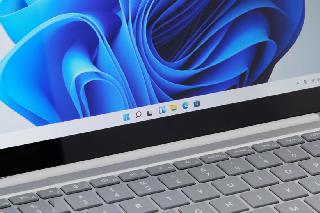